Agrippa Vanities - TIC-80 512 bytes
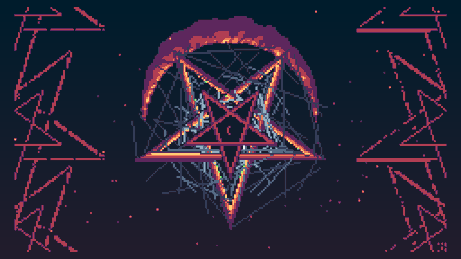
Released in december at Inércia 2023, started out
of boredom in early 2023 from some star polygon
algorithm (with thickness) and as a tribute to black metal, the
initial sketch which was JavaScript didn't satisfy me enough
aesthetically and wasn't animated and i didn't consider a release
at first but kept iterating and eventually converted it to LUA
(better for compression), the first idea to improve the original
sketch was to add some classic demoscene effects related to the
theme so i added a fire effect (common for this
theme) and later the starfield effect (fire sparkles ?) with a
background gradient.
Not a great effort size-wise but i still enjoyed
fighting with the packer/code to polish the end result and add
content, there is many more size optimizations left though, could
perhaps go to 256 bytes with slightly different algorithms (bundled
tris ?) or ordering, there is redundant code right now for the
smaller pentagram and the fire / starfield / overlay effect could
be smaller.
Was silent at first but since it was a black
metal tribute... just couldn't ship it without some buzzing noise
with code i borrowed from my Chmmr intro and
added one week before the party.
Lua code packed with pakettic.
Anecdote : The moon symbol at the center was
added as a filler, thought it looked cool and didn't add much
bytes, curiously found out a bit later that the moon symbol was
also at the center of the pentagram on Man inscribed in a
pentagram from Heinrich Cornelius Agrippa's
De occulta philosophia
libri tres, i also added ORZ signature as Dotsies for
fun, the program without the moon symbol and signature is ~470
bytes.
Forgot to hide the TIC-80 cursor but there is
bytes left to do this without any other changes, there is also bits
of green on the pentagram outline that i don't like, noticed this
much later, it can be fixed easily though.
f=.31415
--{
b={}
c={}
n=f*4
--}
for i=0,3e4 do
--{
b[i]=0
c[i]=.02+math.random()*.1
--}
end
function q(e,s,m,p)ab
--{
line(120+e*math.cos(s),68+e*math.sin(s),120+e*math.cos(m),68+e*math.sin(m),p)
poke(65455+e,64+e%6)
--}
end
SCN=function(e) poke(16320,e/4) end
TIC=function()
cls()
-- fire seed
for i=90,269 do
b[120+48*math.sin(i/58)//1+(68+48*math.cos(i/58))//1*240]=math.random(8)
end
for i=0,5 do
-- big penta
for e=-40,40 do
q(e/8+50,i*n+f,(i+2)*n+f,3-e/20)
end
-- chaos lines
for e=1,120,6 do
q(e/2,i*n+f,(i+2+e)*n*e/4+f*e/8,12+e/28)
end
end
-- small penta
for i=0,5 do
for e=1,29 do
q(e,i*n+f,(i+2)*n+f,16)
end
end
-- black penta with heavy weight (hiding chaos lines)
for i=0,5 do
for e=24,27 do
q(e,i*n+f,(i+2)*n+f,4-e/13)
end
end
-- penta overlay (sides)
for y=0,135 do
for x=70,169 do
if pix(x,y)>0 and pix(x,y)<2 then
--{
pix((x+120)%240,y%136,2)
pix((x+120)%240,(y+64)%136,2)
--}
end
end
end
-- fire
for i=1,15360 do
b[i]=(b[i-1]+b[i+240]+b[i+240+1]+b[i+480])*.23
a=peek4(i)==0 and poke4(i,b[i])
end
-- starfield
for i=0,240,4 do
z=c[i+3]
--{
x=c[i]/z
y=c[i+1]/z
c[i+3]=z-c[i+2]
--}
z=x*x+y*y/3>.17 and pix(120+x*120,68+y*68,z%5)
if x>1 or y>1 then
--{
c[i]=math.random()*5-2
c[i+1]=math.random()*5-2
c[i+3]=4
--}
end
end
--{
print("(",118,66,2)
pix(235,131,2)
pix(235,135,2)
pix(237,131,2)
pix(237,133,2)
pix(237,134,2)
pix(239,131,2)
pix(239,132,2)
pix(239,134,2)
pix(239,135,2)
--}
end
Versions
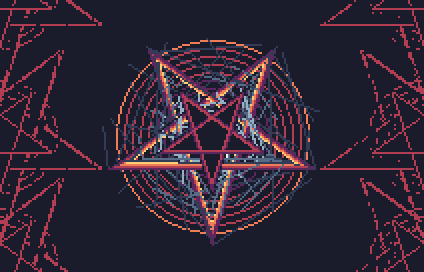
initial version, didn't like the circles
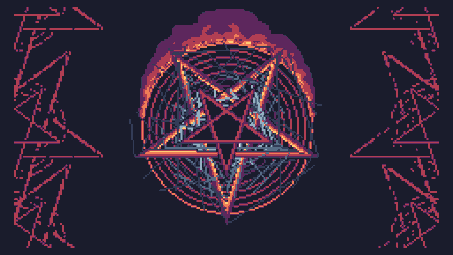
slightly better animated but still felt it was
missing something
Pentagram
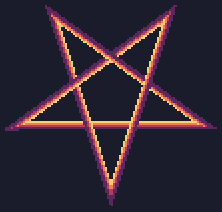
The code that construct the thick pentagram geometry, it all
started from this :
u=math
l=u.cos
h=u.sin
f=.31415
n=f*4
d=240
g=d/2
function q(e,s,m,p)
line(g+e*l(s),68+e*h(s),g+e*l(m),68+e*h(m),p)
end
TIC=function()
cls(0)
for i=0,4 do -- penta
s=i*n+f
for e=-40,40 do -- thickness
q(e/8+50,s,(i+2)*n+f,3-e/20)
end
end
end
Starfield
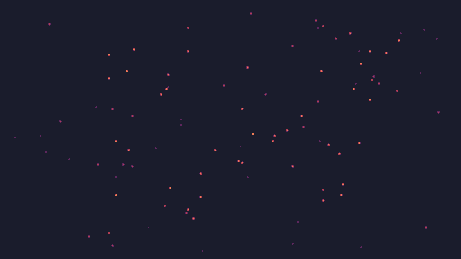
classic
demo effect, filtered in my case to only show stars outside of
a black disk :
if x*x+y*y/3>0.17 then plot...
end
d=240
c={}
g=d/2
for i=0,504 do
c[i]=0.02+math.random()*0.1
end
TIC=function()
cls(0)
for i=0,500,4 do
z=c[i+3]
x=c[i]/z
y=c[i+1]/z
c[i+3]=z-c[i+2]
pix(g+x*g,68+y*68,z%5)
if x>1 or y>1 then
c[i]=math.random()*5-2.4
c[i+1]=math.random()*5-2.4
c[i+3]=4
end
end
end
Fire effect
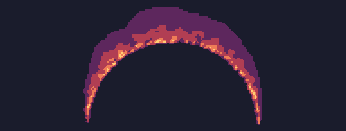
Another classic
demo effect which is related to Poisson's
Equation, a directed diffusion (blur) of values across a grid,
seed values here are randomly chosen on half of a circle at each
frames, the diffusion is done through a lookup of 4 values for
every pixels and scaling down the combined values so it goes toward
0 which correspond to the background color.
On my version the fire effect is behind some other effects so
i also needed a conditional to only plot on background areas,
different layer ordering could also works perhaps but i also read
the framebuffer to show the edges overlay so i don't know how it
would work out.
d=240
b={}
g=d/2
for i=0,3e4 do
b[i]=0
end
cls(0)
TIC=function()
for i=90,269 do
b[g+48*math.sin(i/58)//1+(68+48*math.cos(i/58))//1*d]=math.random(8)
-- half-circle random
end
for y=0,64 do
for x=1,d-1 do
-- diffusion (note : replaced with poke4 / peek4 +
single loop on the final release)
b[x+y*d]=(b[x+y*d-1]+b[x+y*d+d]+b[x+y*d+d+1]+b[x+y*d+d+d])*.235
-- plot on screen
pix(x,y,b[x+y*d])
end
end
end
Aside : Black metal
Black
metal music is something i engaged with as a teenager in the
early 2000s (after Rock and Nu metal and before
delving into Electronic
music and Jazz) with bands such as
Dimmu
Borgir, Bathory or
Arcturus, i was
not really interested by the lyrics (i rarely care about lyrics at
first) but i guess i was impressed by the seemingly general
irreverence, the energy and profane symbolism, all good fuel for
teenage rebellion, the moody romanticism was
also important to me as i was favoring music with high keyboards
emphasis. This period is also when i discovered the
demoscene.
Best example of these years was perhaps moody tracks such as
For all tid
(always likened the drums pattern as some kind of trot), always liked these
cheesy preset keyboards intro independently of any metal
genres. (dungeon synths which carry these keyboards into full
length tracks strangely bore me though with some exceptions such as
early Mortiis
or Summoning)
The satanic theme wasn't of any interest to me beyond the
edginess of it, i didn't care much about it and still don't beyond
what is now curiosity, i am pretty much agnostic sometimes leaning
to atheism with a Spinoza view, i was never accustomed to any
religious ideas whatsoever in my life.
The satanic theme re-framed into a theistic Gnostic /
dualist cosmogony (could also fit pantheism) pushed by some black
metal bands (eg: Abigor on
Time Is The Sulphur In The Veins Of The Saint - An Excursion on
Satan's Fragmenting Principle (2010) for example, this
interpretation is loosely based on the lyrics but i also heard some
of this in Brigitte
Fontaine Hermaphrodite
song, Coil
Blood from
the air or even in Jean Genet works),
this view devoid of any liturgy nor rituals is something
interesting to me, i see it as some sort of extreme spiritual
extension (much wider in scope) of the sort of principles that one
can find in anarchism (pursuit of personal knowledge, liberation
from oppressive forces, high skepticism etc.), all the paradoxes
that this theme spawn could fuel and resolve to a sort of mythology
/ cosmogony with the commonly seen biblical figures (reversed)
referring to man (actually all that exist) and whatever system of
oppression he (whatever 'he' is here) is currently in (so demiurge
is the keeper of that structure ?), in this case the best state is
non existence i guess which can be considered one of the higher
realm;
primal chaos, existence can be seen as an everlasting try
(rebellion) to reach that state even if briefly (for those who
knows i guess ?), it perhaps always "fall" through though (never
ending falls, never works), singularities, the true god is
something abstract (fragmented ?) and self referential for anything
that exist, it may actually refer to one self across time and
fields of possibles, it also means that believing it is
actually believing in one self, we are one
(which is probably a reference to the first principle which i guess
refer to the first law of thermodynamics which state that energy
can only be altered, neither created nor destroyed), the Gnosis of the Gnostic (a
trail, bottle message) lie in everything that exist (more or less)
as some sort of leitmotiv to catch on.
Time may also have a special meaning : Time exists so that everything doesn’t happen at once. so
time would actually be the mechanism by which rebellion, salvation
is realized (Gnosis), if everything
would happen at once there would be perpetual servitude (immediate
and total subjugation to oppressive structures), the illusion of
time (?) is a fragmented way to avoid and realize this (assemble;
through experience ?).
Another interpretation of Gnosticism that i like
without the cosmic and mythological bullshit (so metaphorical and
allegorical) could be that the demiurge actually represent whatever
system of oppression is in exercise for an individual, the material
world in this idea represent the structure itself (power
structures) and whatever enforcement it does to the individual
directly or indirectly, even though it may appear as benevolent it
is actually largely ignorant and perhaps completely misguided,
filled with false "gods" while the non-material (and
unknowledgeable) world is "good" because it stay unrealized, full
of possibles, in this view i like to think that the Gnosis is just
whatever someone can infer from all the scattered knowledge to go
past the "enslavement" and thus approach the non-material world
without ever materializing anything from it (because it may shift
into oppression), i find this quite funny because it interpret
Gnosticism as a distilled disguised criticism of whatever enforce
rules over individuals and a pacific way to go past that through
introspection, individual knowledge, understanding, criticizing and
experiencing, solitary seekers in this framework make
sense.
All this is probably farfetched edgy individual
interpretation (aka bullshit) though and should be highly
questioned and disregarded; just had some quick interpretation fun
after listening to some music. I guess that the interesting bits i
draw from this is that some of the essence of what is talked on in
black metal has close ties with anarchism and its values in some
ways (i see some of this in the demoscene as well), rebellion from
the status quo, personal freedom, black metal has punk roots so no
wonder, it is known there is overlapping ideas there, most of the
ideas doesn't draw from organized religions / coercive authority
but from earlier forms that existed prior it which were less
dogmatic, free form and wilder, such ideas were often severely
punished by history through the implementation of heresy and
orthodoxy; metal music also draw from all kinds of outsider stories
such as witches in Bathory For all those who
died which was inspired by a Erica Jong poem, it
is outsider music at heart.
A fun (mostly related) essay on Gnosticism in metal :
Of chaos and internal fire: the quest for nothingness by lyrical
manifestations of re-interpreted Gnostic thought
back to top


