Apollonian - Linux 256 bytes
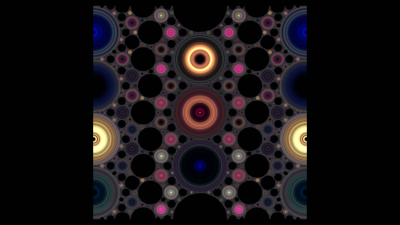
Still C code and still Minsky circle, this draw some
kind of Apollonian
gasket fractal, not many big technical improvements there
except colors tweak and clearer structure, it use the downscale
pass i used in QuasiSpace to
avoid bounds checking.
It can be seen as an improved version of QuasiSpace,
the code is pretty similar ! Could probably be done in 128 bytes on
Linux by doing it in full assembly perhaps even C...
The algorithm is (roughly) as follow (you can "fill" the
inside of the circles by adjusting x and y outside the loop)
:
// init
x = 0
y = 1
// loop, preferably iterating more than once per loop
x -= (y << 2)
y -= (x >> 5) - (x >> 1)
x += (x >> 15) - (y << 2)
// scale it down when you plot it (here for a 512x512 image) : (x
>> 23), (y >> 23)
// outside the loop adjust x,y by adding the frame step (scaled up
by a big factor) to "fill" in details
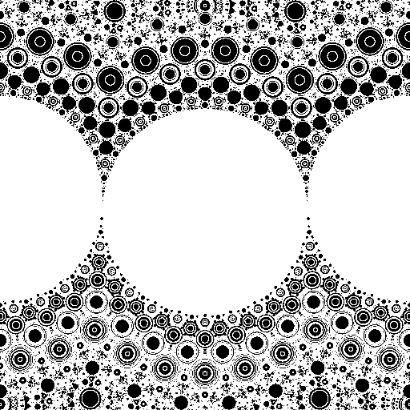
adjusting x and y outside the loop "fill" in
details
Twitter variant
setup=_=>{createCanvas(512,512);x=y=1e18;for(i=6e6;i--;){x-=y<<3;y-=(x>>8)-(x>>2);x+=(x>>18)-(y<<3);point(x>>22,y>>22)}}
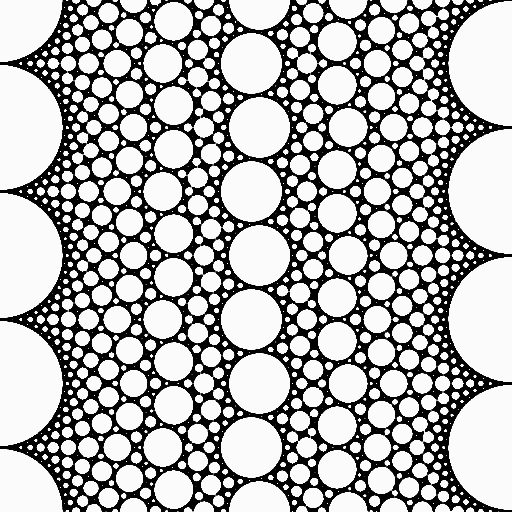
variant published on Twitter (p5js
framework)
More variants
Interesting variations can be done by adjusting the offsets
such as :
// init
x = 0
y = 0
// in loop, preferably iterating more than once per loop
x += (y >> 2) + (1 << 27)
y -= (x >> 1)
x += (y >> 2) - (1 << 31)
// scale it down when you plot it (here for a 512x512 image) : (x
>> 23), (y >> 23)
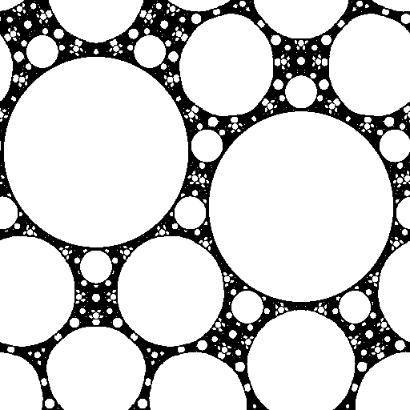
adjusting offsets (dissimilar for first x and
second x)
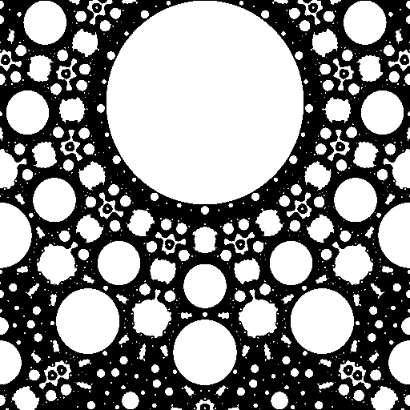
with same offset value for both x, probably
similar to Trinskys
This also works with floats although values for x
and y should be bounded (modulo) to produce the patterns, using
floating
point is flexible and may produce better results.
// init
x = 0
y = 0
for (let i = 0; i < 1e4; i += 1) {
x += (y * 0.25) + (1 << 29)
x %= (1 << 31) // necessary for floats for the
patterns to appear (will be the standard Minsky circle
otherwise)
y -= (x * 0.5)
y %= (1 << 31)
x += (y * 0.25) + (1 << 30)
x %= (1 << 31)
// scale it down when you plot it (here for a 512x512 image)
: (x >> 22), (y >> 22)
}
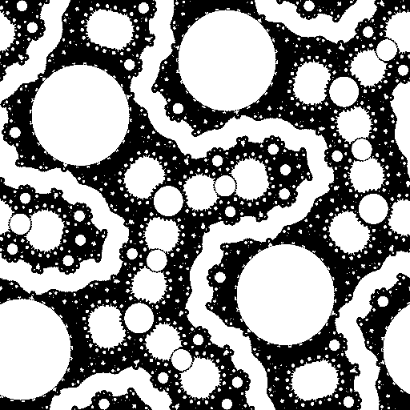
with floats
back to top


